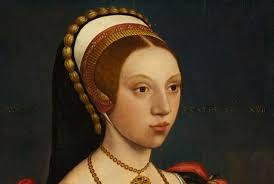
To position objects in defined spatial relationships presupposes a clear understanding of the involved coordinate systems. Unfortunately, several systems with several conventions are involved: DOM & CSS, SVG, Canvas
We need a common reference system to switch between these coordinate systems. As the uttermost context, the browser page coordinate system is the most natural one. A simple API was long missing but has now been established in most modern browsers with window.convertPointFromNoteToPage and the inverse window.convertPointFromPageToNode. Although MDN Web Docs warns about their Non-standard nature the methods work in browsers targeted by the IWM Browser project. This doctest assures that this assumption can be tested.
Let's look at a scatter object with a rotatable local coordinate system. We try to follow a point in this local coordinate system by showing a marker outside the scatter that follows the point.